Quick access to articles on this page:
more on the next page...
the Threat Model for BGP Path Security document lists, as RFCs usually do, relevant terms with their respective definitions. It can be a quick way to get an understanding of these abbreviations you often come across but never dare to google:
-
Autonomous System (AS): An AS is a set of one or more IP networks operated by a single administrative entity.
-
AS Number (ASN): An ASN is a 2- or 4-byte number issued by a registry to identify an AS in BGP.
-
Border Gateway Protocol (BGP): A path vector protocol used to convey "reachability" information among ASes in support of inter-domain routing.
-
False (Route) Origination: If a network operator originates a route for a prefix that the operator does not hold (and that has not been authorized to originate by the prefix holder), this is termed false route origination.
-
Internet Service Provider (ISP): An organization managing (and typically selling) Internet services to other organizations or individuals.
-
Internet Number Resources (INRs): IPv4 or IPv6 address space and ASNs.
-
Internet Registry: An organization that manages the allocation or distribution of INRs. This encompasses the Internet Assigned Number Authority (IANA), Regional Internet Registries (RIRs), National Internet Registries (NIRs), and Local Internet Registries (LIRs) (network operators).
-
Network Operator: An entity that manages an AS and thus emits (E)BGP updates, e.g., an ISP.
-
Network Operations Center (NOC): A network operator employs a set of equipment and a staff to manage a network, typically on a 24/7 basis. The equipment and staff are often referred to as the NOC for the network.
-
Prefix: A prefix is an IP address and a mask used to specify a set of addresses that are grouped together for purposes of routing.
-
Public Key Infrastructure (PKI): A PKI is a collection of hardware, software, people, policies, and procedures used to create, manage, distribute, store, and revoke digital certificates.
-
Relying Parties (RPs): An RP is an entity that makes use of signed products from a PKI, i.e., it relies on signed data that is verified using certificates and Certificate Revocation Lists (CRLs) from a PKI.
-
RPKI Repository System: The RPKI repository system consists of a distributed set of loosely synchronized databases.
-
Resource PKI (RPKI): A PKI operated by the entities that manage INRs and that issue X.509 certificates (and CRLs) that attest to the holdings of INRs.
-
RPKI Signed Object: An RPKI signed object is a data object encapsulated with Cryptographic Message Syntax (CMS) that complies with the format and semantics defined in [RFC6488].
-
Route: In the Internet, a route is a prefix and an associated sequence of ASNs that indicates a path via which traffic destined for the prefix can be directed. (The route includes the origin AS.)
- Route Leak: A route leak is said to occur when AS-A advertises routes that it has received from AS-B to the neighbors of AS-A, but AS-A is not viewed as a transit provider for the prefixes in the route.
In cryptography, zeroisation (also spelled zeroization) is the practice of erasing sensitive parameters (electronically stored data, cryptographic keys, and CSPs) from a cryptographic module to prevent their disclosure if the equipment is captured. This is generally accomplished by altering or deleting the contents to prevent recovery of the data. When encryption was performed by mechanical devices, this would often mean changing all the machine's settings to some fixed, meaningless value, such as zero. On machines with letter settings rather than numerals, the letter 'O' was often used instead. Some machines had a button or lever for performing this process in a single step. Zeroisation would typically be performed at the end of an encryption session to prevent accidental disclosure of the keys, or immediately when there was a risk of capture by an adversary.
from Wikipedia
The Mcgrew draft (on the old stateful signature scheme LMS) has a section on "how not to mess with implementing a stateful scheme". It's pretty scary.
The LMS signature system, like all N-time signature systems, requires
that the signer maintain state across different invocations of the
signing algorithm, to ensure that none of the component one-time
signature systems are used more than once. This section calls out
some important practical considerations around this statefulness.
In a typical computing environment, a private key will be stored in
non-volatile media such as on a hard drive. Before it is used to
sign a message, it will be read into an application's Random Access
Memory (RAM). After a signature is generated, the value of the
private key will need to be updated by writing the new value of the
private key into non-volatile storage. It is essential for security
that the application ensure that this value is actually written into
that storage, yet there may be one or more memory caches between it
and the application. Memory caching is commonly done in the file
system, and in a physical memory unit on the hard disk that is dedicated to that purpose. To ensure that the updated value is
written to physical media, the application may need to take several
special steps. In a POSIX environment, for instance,the O_SYNC flag
(for the open() system call) will cause invocations of the write()
system call to block the calling process until the data has been to
the underlying hardware. However, if that hardware has its own
memory cache, it must be separately dealt with using an operating
system or device specific tool such as hdparm to flush the on-drive
cache, or turn off write caching for that drive. Because these
details vary across different operating systems and devices, this
note does not attempt to provide complete guidance; instead, we call
the implementer's attention to these issues.
When hierarchical signatures are used, an easy way to minimize the
private key synchronization issues is to have the private key for the
second level resident in RAM only, and never write that value into
non-volatile memory. A new second level public/private key pair will
be generated whenever the application (re)starts; thus, failures such
as a power outage or application crash are automatically
accommodated. Implementations SHOULD use this approach wherever
possible.
This post is the ending of a series of blogposts on hash-based signatures. You can find part I here
So now we're getting into the interesting part, the real signatures schemes.
PQCrypto has released an initial recommendations document a few months ago. The two post-quantum algorithms advised there were XMSS and SPHINCS:

This blogpost will be presenting XMSS, a stateful signature scheme, while the next will focus on SPHINCS, the first stateless signature scheme!
XMSS
The eXtended Merkle Signature Scheme (XMSS) was introduced in 2011 and became an internet-draft in 2015.
The main construction looks like a Merkle tree, excepts a few things. The XMSS tree has a mask XORed to the child nodes before getting hashed in their parents node. It's a different mask for every node:
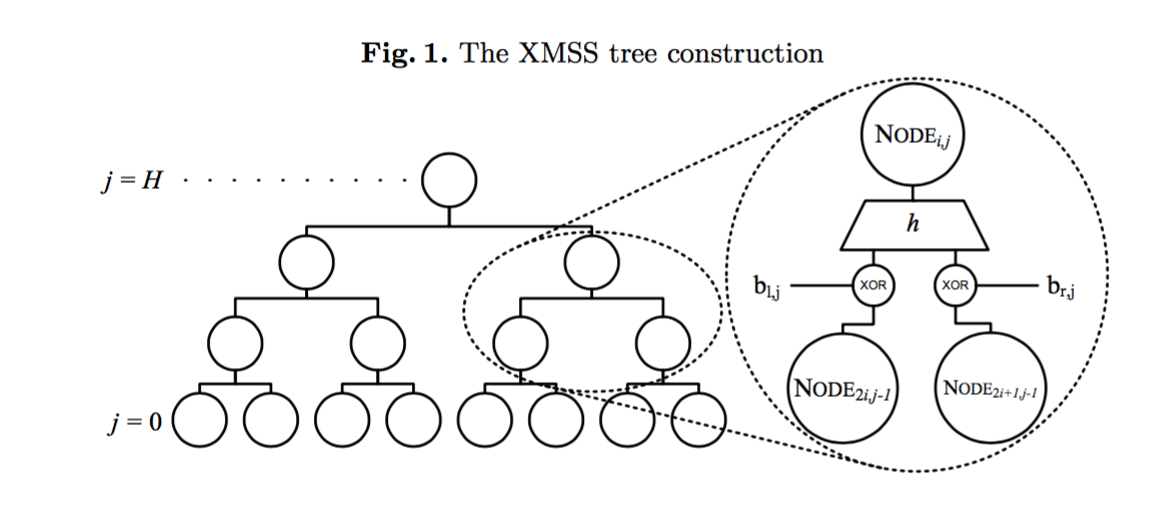
The second particularity is that a leaf of the XMSS tree is not a hash of a one-time signature public key, but the root of another tree called a L-tree.
A L-tree has the same idea of masks applied to its nodes hashes, different from the main XMSS Trees, but common to all the L-trees.
Inside the leaves of any L-tree are stored the elements of a WOTS+ public key. This scheme is explained at the end of the first article of this series.
If like me you're wondering why they store a WOTS+ public key in a tree, here's what Huelsing has to say about it:
The tree is not used to store a WOTS public key but to hash it in a way that we can prove that a second-preimage resistant hash function suffices (instead of a collision resistant one).
Also, the main public key is composed of the root node of the XMSS tree as well as the bit masks used in the XMSS tree and a L-tree.
SPHINCS
SPHINCS is the more recent one, combining a good numbers of advances in the field and even more! Bringing the statelessness we were all waiting for.
Yup, this means that you don't have to keep the state anymore. But before explaining how they did that, let's see how SPHINCS works.
First, SPHINCS is made out of many trees.
Let's look at the first tree:
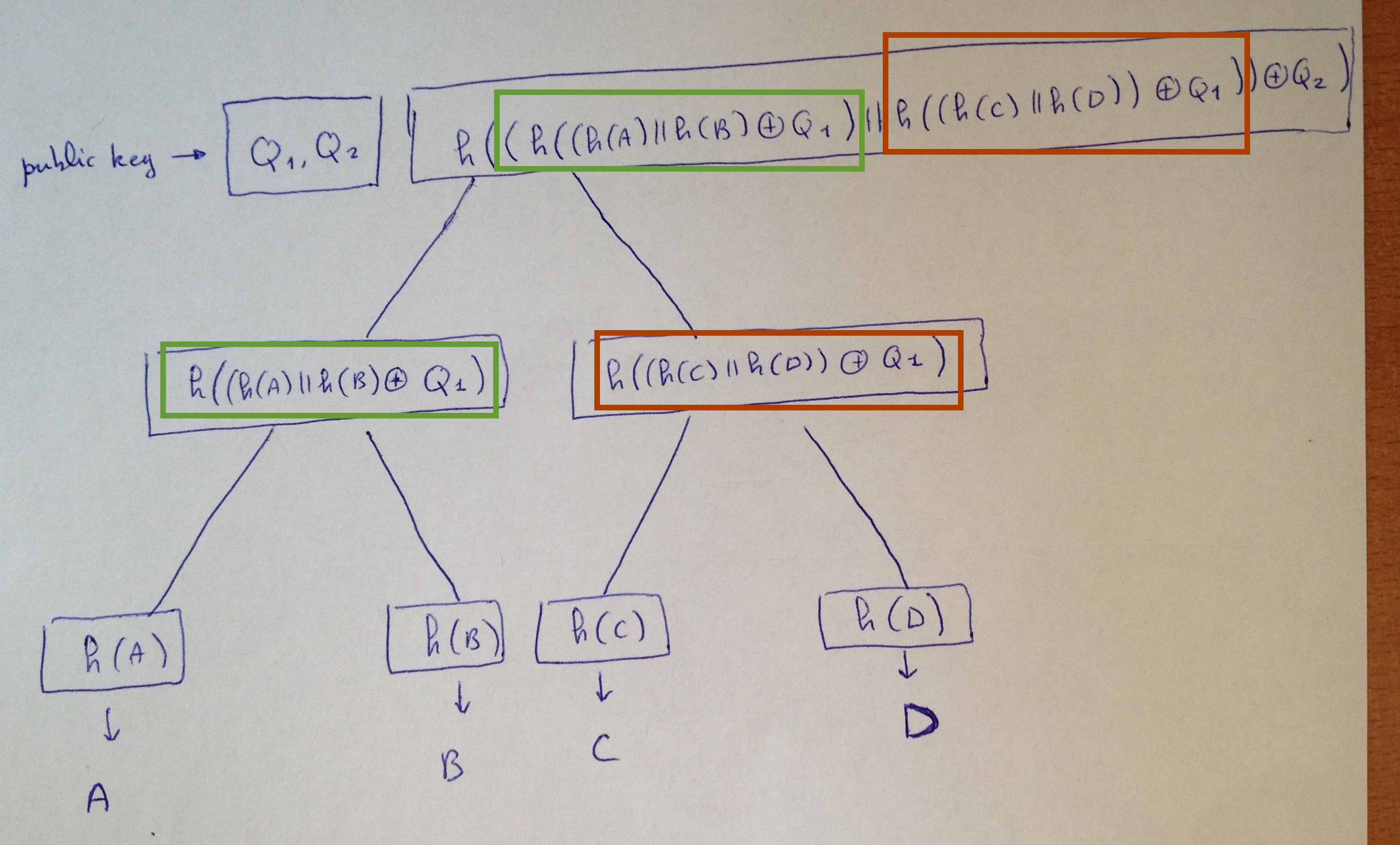
- Each node is the hash of the XOR of the concatenation of the previous nodes with a level bitmask.
- The public key is the root hash along with the bitmasks.
- The leaves of the tree are the compressed public keys of WOTS+ L-trees.
See the WOTS+ L-trees as the same XMSS L-tree we previously explained, except that the bitmask part looks more like a SPHINCS hash tree (a unique mask per level).
Each leaves, containing one Winternitz one-time signature, allow us to sign another tree. So that we know have a second layer of 4 SPHINCS trees, containing themselves WOTS+ public keys at their leaves.
This go on and on... according to your initial parameter. Finally when you reach the layer 0, the WOTS+ signatures won't sign other SPHINCS trees but HORS trees.
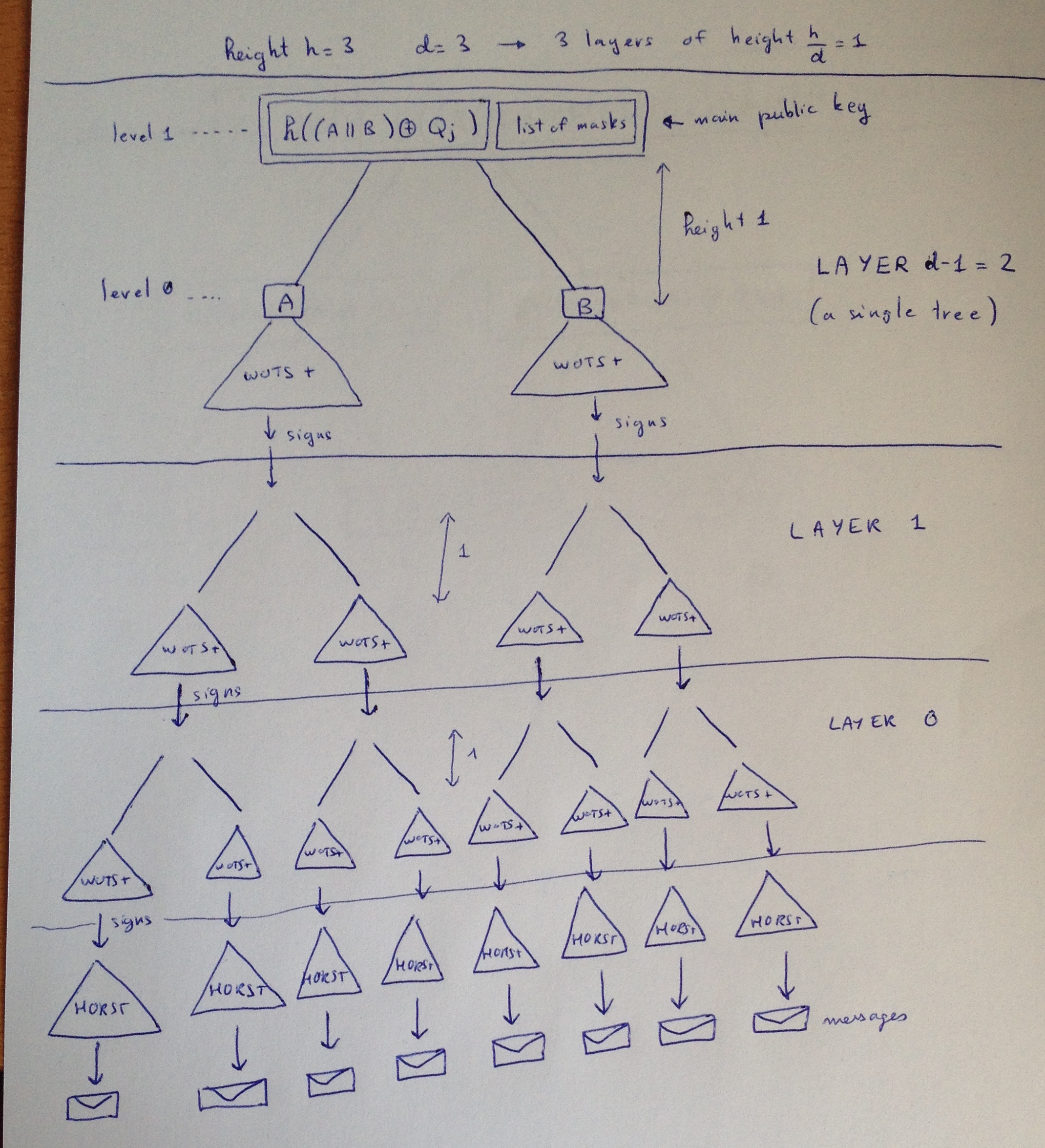
A HORST or HORS tree is the same as a L-tree but this time containing a HORS few-time signature instead of a Winternitz one-time signature. We will use them to sign our messages, and this will increase the security of the scheme since if we do sign a message with the same HORS key it won't be a disaster.
Here's a diagram taken out of the SPHINCS paper making abstraction of WOTS+ L-trees (displaying them as signature of the next SPHINCS tree) and showing only one path to a message.
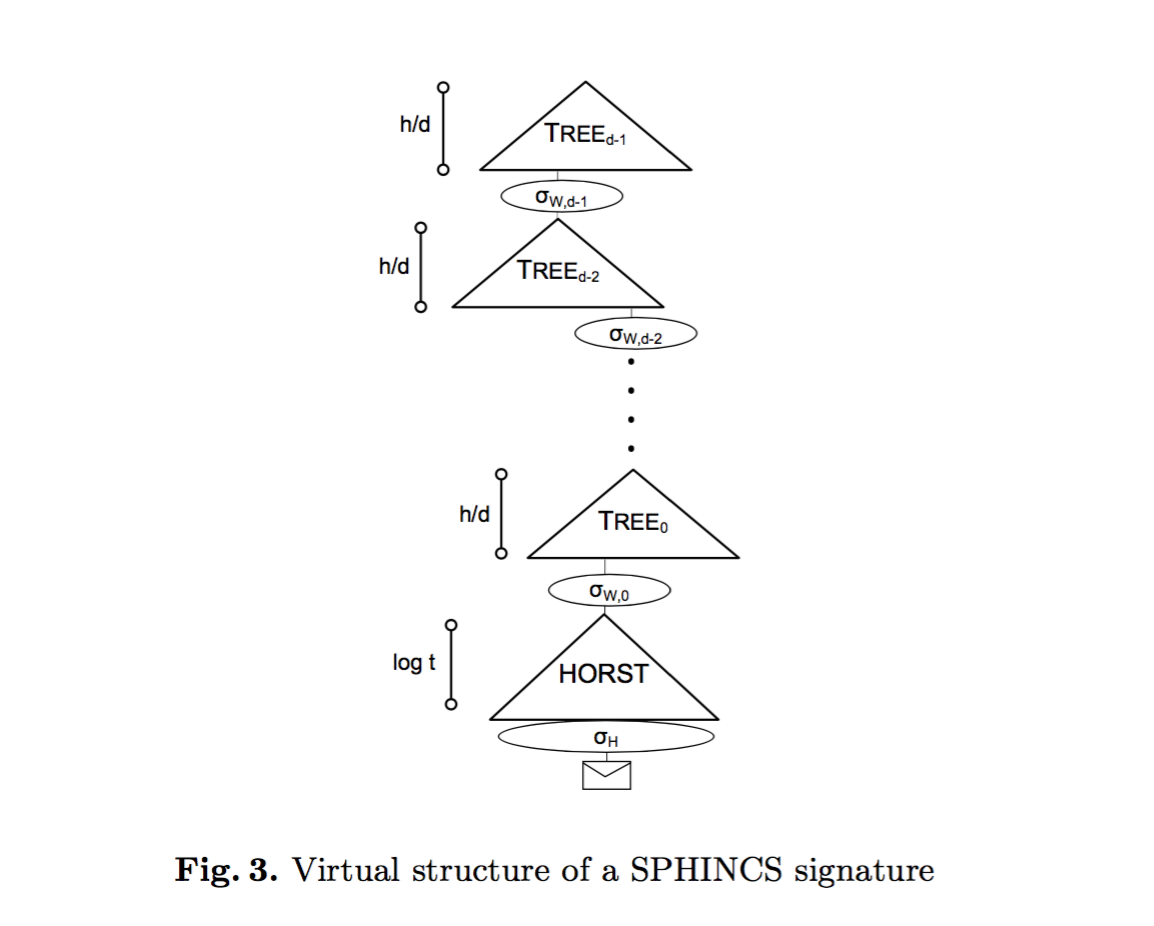
When signing a message M you first create a "randomized" hash of M and a "random" index. I put random in quotes because everything in SPHINCS is deterministically computed with a PRF. The index now tells you what HORST to pick to sign the randomized hash of M. This is how you get rid of the state: by picking an index deterministically according to the message. Signing the same message again should use the same HORST, signing two different messages should make use of two different HORST with good probabilities.
And this is how this series end!
EDIT: here's another diagram from Armed SPHINCS, I find it pretty nice!
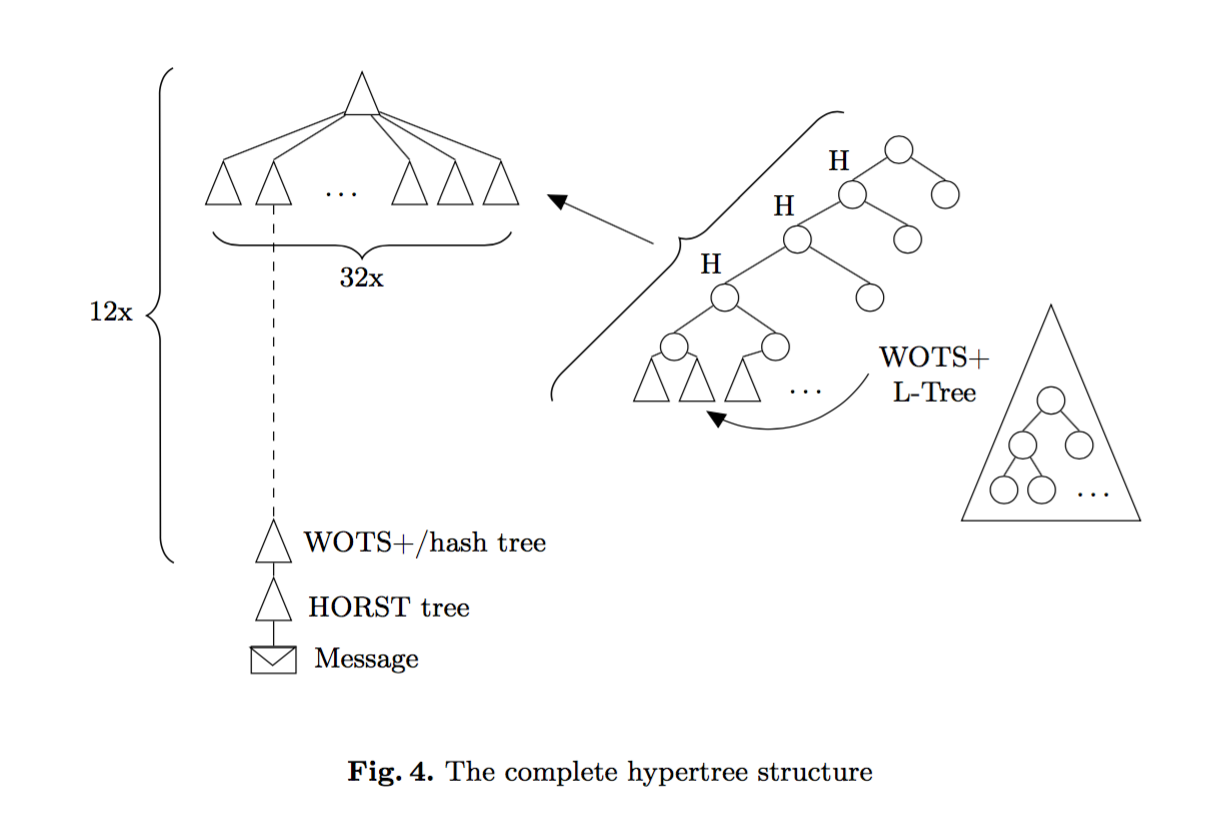
We saw previously what were one-time signatures (OTS), then what were few-time signatures (FTS). But now is time to see how to have practical signature schemes based on hash functions. Signature schemes that you can use many times, and ideally as many times as you'd want.
If you haven't read Part I and Part II it's not necessarily a bad thing since we will make abstraction of those. Just think about OTS as a public key/private key pair that you can only use once to sign a message.
Dumb trees
The first idea that comes to mind could be to use a bunch of one-time signatures (use your OTS scheme of preference). The first time you would want to sign something you would use the first OTS keypair, and then never use it again. The second time you would want to sign something, you would use the second OTS keypair, and then never use it again. This can get repetitive and I'm sure you know where I'm going with this. This would also be pretty bad because your public key would consist of all the OTS public keys (and if you want to be able to use your signature scheme a lot, you will have a lot of OTS public keys).
One way of reducing the storage amount, secret-key wise, is to use a seed in a pseudo-random number generator to generate all the secret keys. This way you don't need to store any secret-key, only the seed.
But still, the public key is way too large to be practical.
Merkle trees
To link all of these OTS public keys to one main public keys, there is one very easy way, it's to use a Merkle tree. A solution invented by Merkle in 1979 but published a decade later because of some uninteresting editorial problems.
Here's a very simple definition: a Merkle tree is a basic binary tree where every node is a hash of its childs, the root is our public key and the leaves are the hashes of our OTS public keys. Here's a drawing because one picture is clearer than a thousand words:
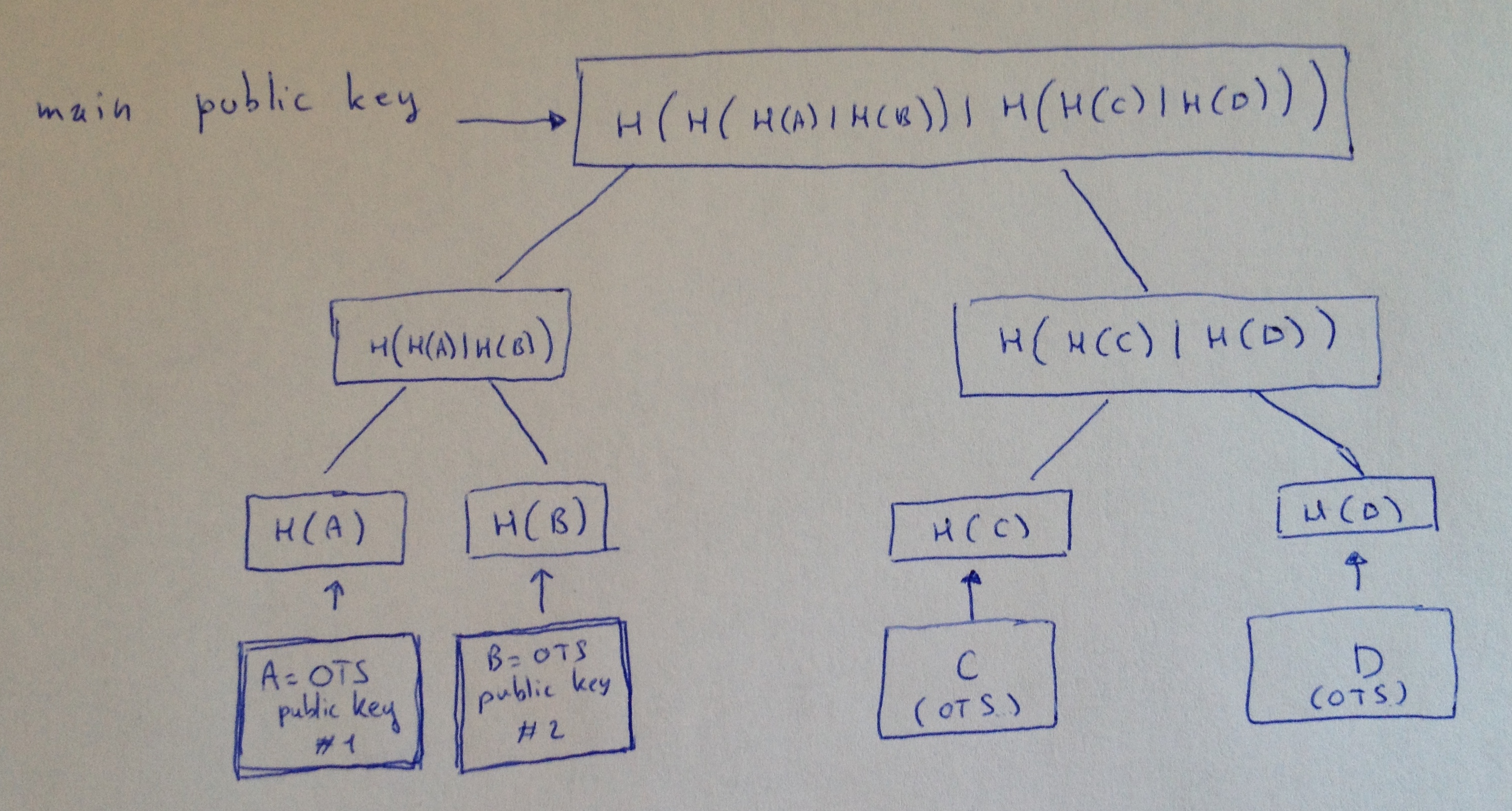
So the first time you would use this tree to sign something: you would use the first OTS public key (A), and then never use it again. Then you would use the B OTS public key, then the C one, and finally the D one. So you can sign 4 messages in total with our example tree. A bigger tree would allow you to sign more messages.
The attractive idea here is that your public key only consist of the root of the tree, and every time you sign something your signature consists of only a few hashes: the authentication path.
In our example, a signature with the first OTS key (A) would be: (1, signature, public key A, authentication path)
-
1 is the index of the signing leaf. You have to keep that in mind: you can't re-use that leaf's OTS. This makes our scheme a stateful scheme.
-
The signature is our OTS published secret keys (see the previous parts of this series of articles).
-
The public key is our OTS public key, to verify the signature.
- The authentication path is a list of nodes (so a list of hashes) that allows us to recompute the root (our main public key).
Let's understand the authentication path. Here's the previous example with the authentication path highlighted after signing something with the first OTS (A).
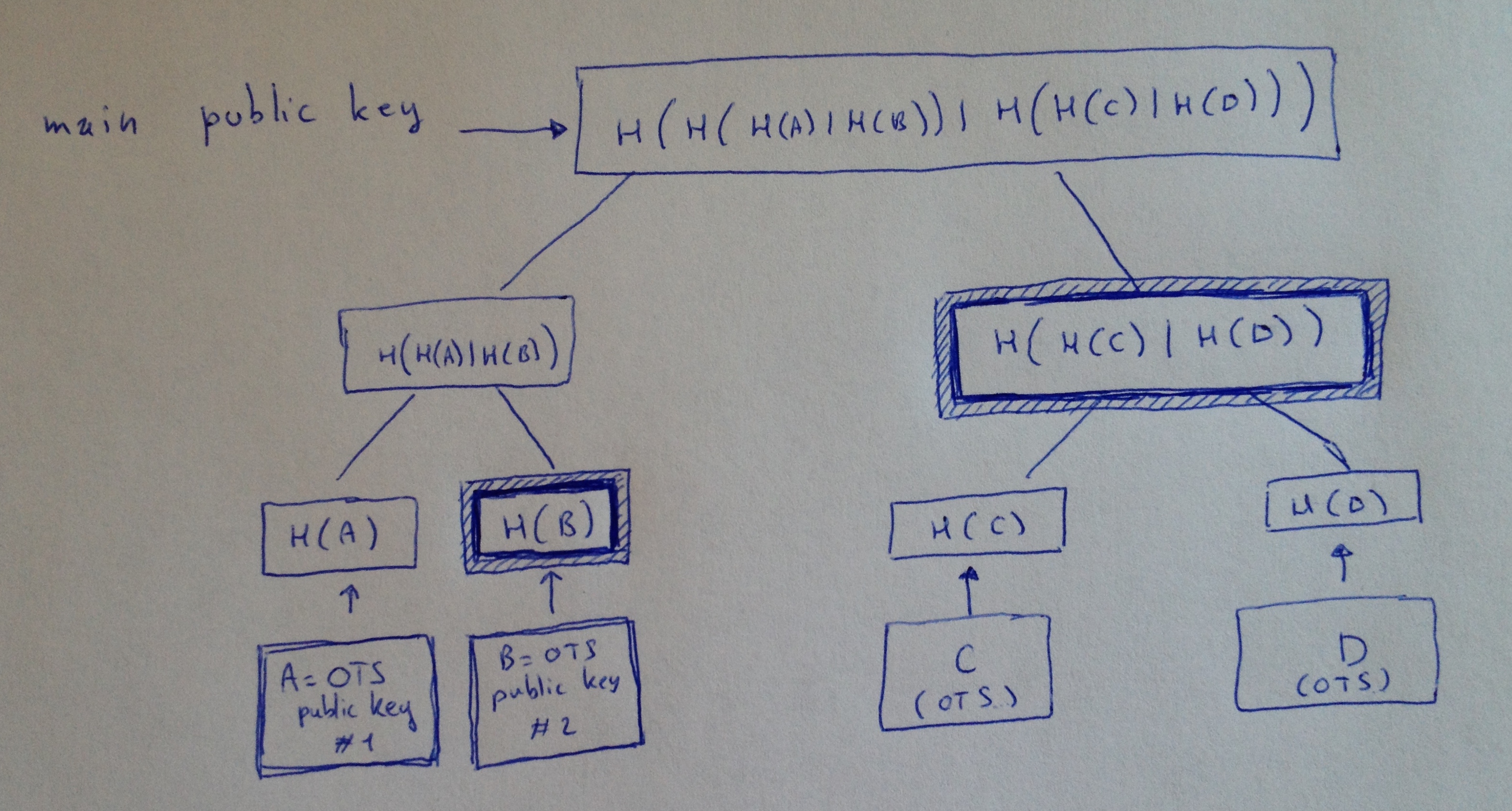
We can see that with our OTS public key, and our two hashes (the neighbor nodes of all the nodes in the path from our signing leaf to the root) we can compute the main public key. And thus we can verify that this was indeed a signature that originated from that main public key.
Thanks to this technique we don't to know all of the OTS public keys to verify that main public key. This saves space and computation.
And that's it, that's the simple concept of the Merkle's signature scheme. A many-times signature scheme based on hashes.
...part IV is here
If you missed the previous blogpost on OTS, go check it out first. This is about a construction a bit more useful, that allows to sign more than one signature with the same small public-key/private-key. The final goal of this series is to see how hash-based signature schemes are built. But they are not the only applications of one-time signatures (OTS) and few-times signatures (FTS).
For completeness here's a quote of some paper about other researched applications:
One-time signatures have found applications in constructions of ordinary signature schemes [Mer87, Mer89], forward-secure signature schemes [AR00], on-line/off-line signature schemes [EGM96], and stream/multicast authentication [Roh99], among others
[...]
BiBa broadcast authentication scheme of[Per01]
But let's not waste time on these, today's topic is HORS!
HORS
HORS comes from an update of BiBa (for "Bins and Balls"), published in 2002 by the Reyzin father and son in a paper called Better than BiBa: Short One-time Signatures with Fast Signing and Verifying.
The first construction, based on one-way functions, starts very similarly to OTS: generate a list of integers that will be your private key, then hash each of these integers and you will obtain your public key.
But this time to sign, you will also need a selection function \(S\) that will give you a list of index according to your message \(m\). For the moment we will make abstraction of it.
In the following example, I chose the parameters \(t = 5\) and \(k = 2\). That means that I can sign messages \(m \) whose decimal value (if interpreted as an integer) is smaller than \( \binom{t}{k} = 10 \). It also tells me that the length of my private key (and thus public key) will be of \( 5 \) while my signatures will be of length \( 2 \) (the selection function S will output 2 indexes).
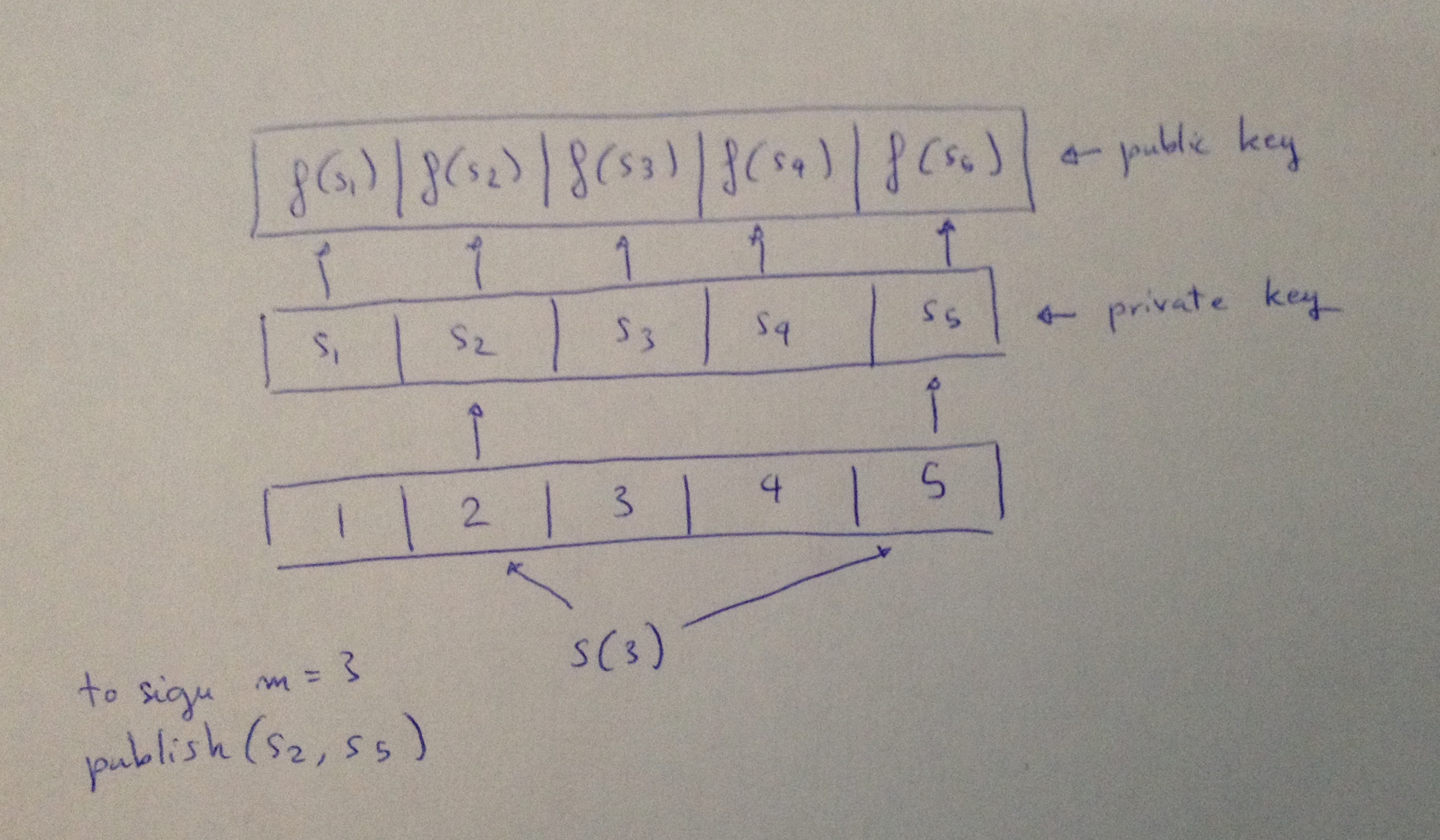
Using a good selection function S (a bijective function), it is impossible to sign two messages with the same elements from the private key. But still, after two signatures it should be pretty easy to forge new ones.
The second construction is what we call the HORS signature scheme. It is based on "subset-resilitient" functions instead of one-way functions. The selection function \(S\) is also replaced by another function \(H\) that makes it infeasible to find two messages \(m_1\) and \(m_2\) such that \(H(m_2) \subseteq H(m_1)\).
More than that, if we want the scheme to be a few-times signature scheme, if the signer provides \(r\) signatures it should be infeasible to find a message \(m'\) such that \(H(m') \subseteq H(m_1) \cup \dots \cup H(m_r) \). This is actually the definition of "subset-resilient". Our selection function \(H\) is r-subset-resilient if any attacker cannot find (even with small probability), and in polynomial time, a set of \(r+1\) messages that would confirm the previous formula. From the paper this is the exact definition (but it basically mean what I just said)
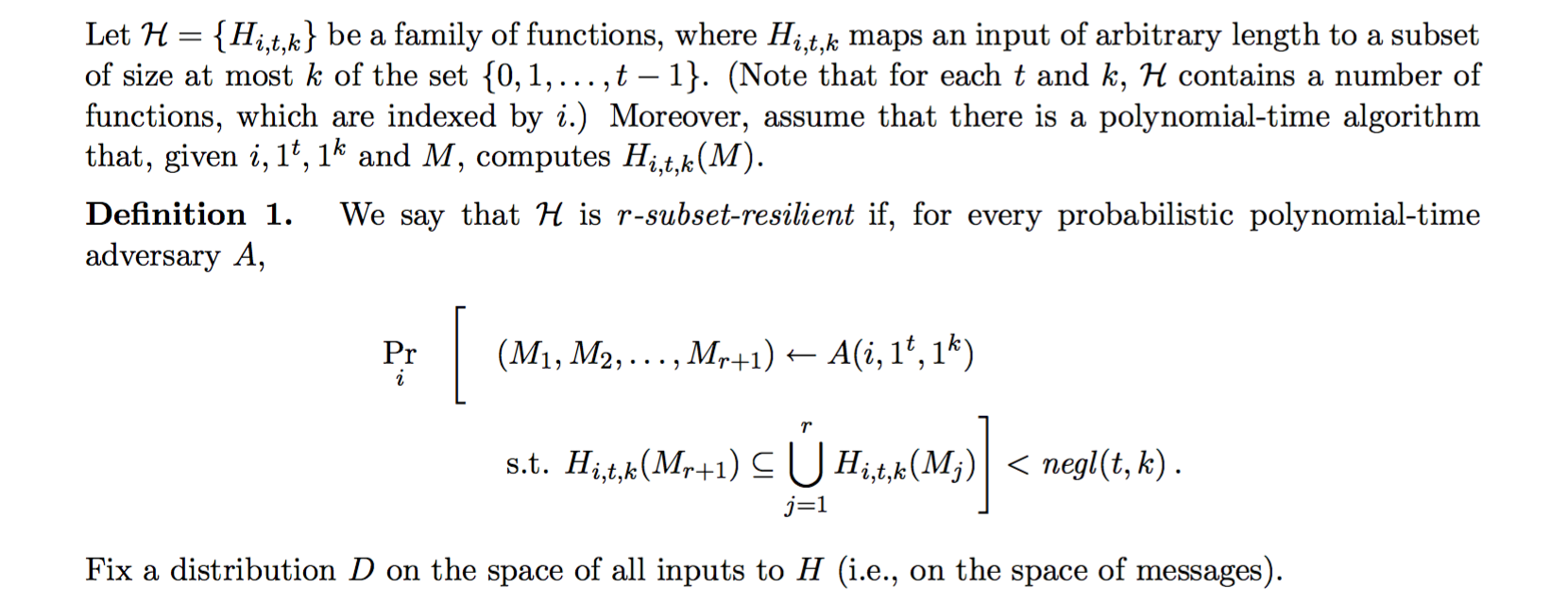
so imagine the same previous scheme:
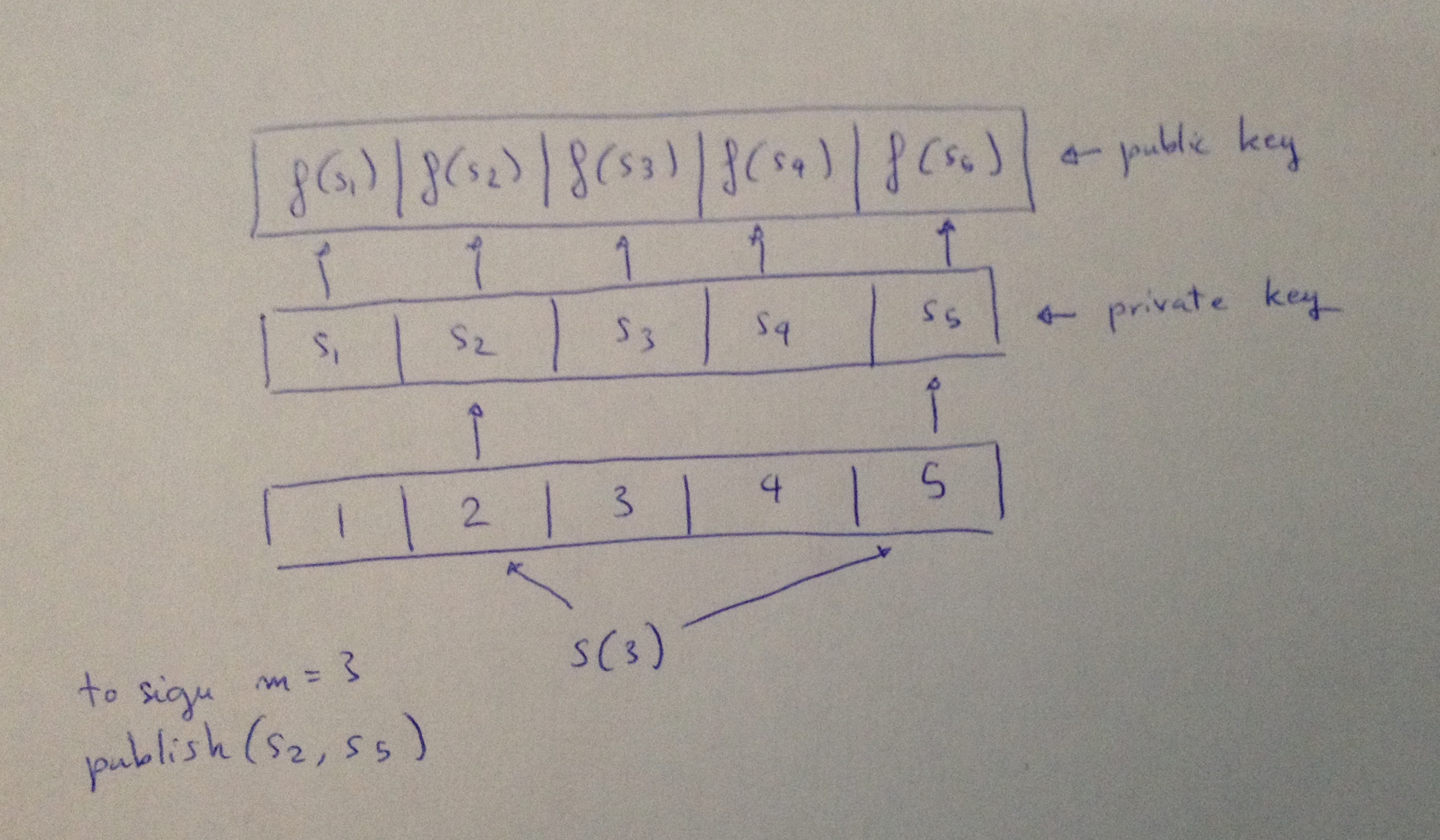
But here the selection function is not a bijection anymore, so it's hard to reverse. So knowing the signatures of a previous set of message, it's hard to know what messages would use such indexes.
This is done in theory by using a random oracle, in practice by using a hash function. This is why our scheme is called HORS for Hash to Obtain Random Subset.
If you're really curious, here's our new selection function:
to sign a message \(m\):
-
\(h = Hash(m)\)
-
Split \(h\) into \(h_1, \dots, h_k\)
-
Interpret each \(h_j\) as an integer \(i_j\)
- The signature is \( sk_{i_1}, \dots, sk_{i_k} \)
And since people seem to like my drawings:
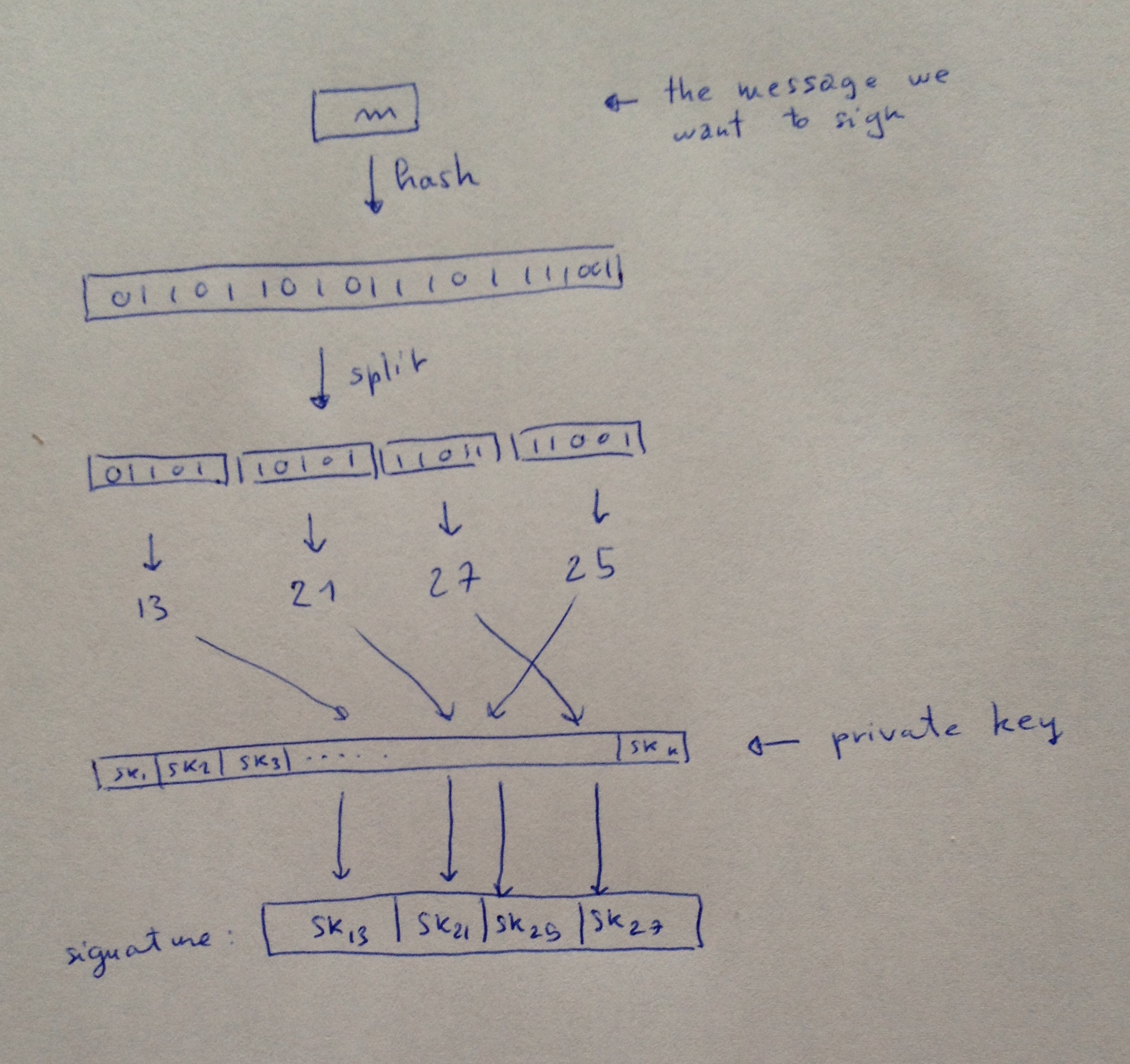
...Part III is here
Lamport
On October 18th 1979, Leslie Lamport published his concept of One Time Signatures.
Most signature schemes rely in part on one-way functions, typically hash functions, for their security proofs. The beauty of Lamport scheme was that this signature was only relying on the security of these one-way functions.
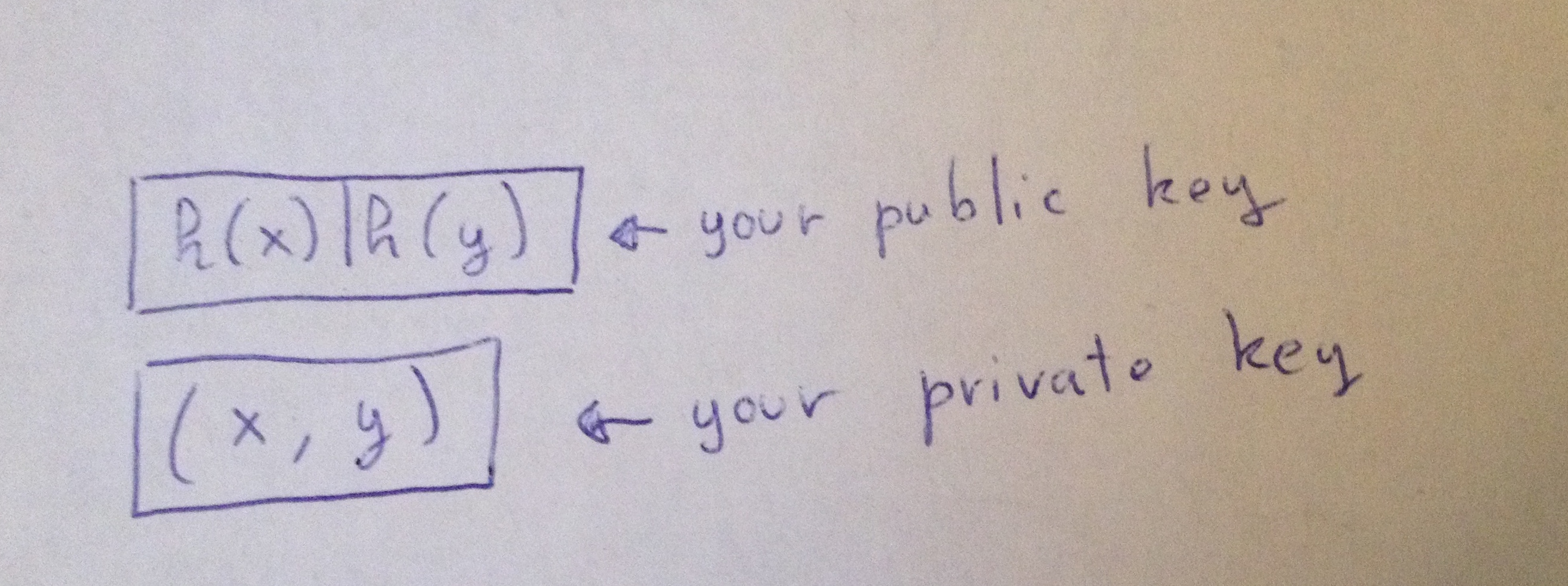
here you have a very simple scheme, where \(x\) and \(y\) are both integers, and to sign a single bit:
Pretty simple right? Don't use it to sign twice obviously.
Now what happens if you want to sign multiple bits? What you could do is hash the message you want to sign (so that it has a predictible output length), for example with SHA-256.
Now you need 256 private key pairs:
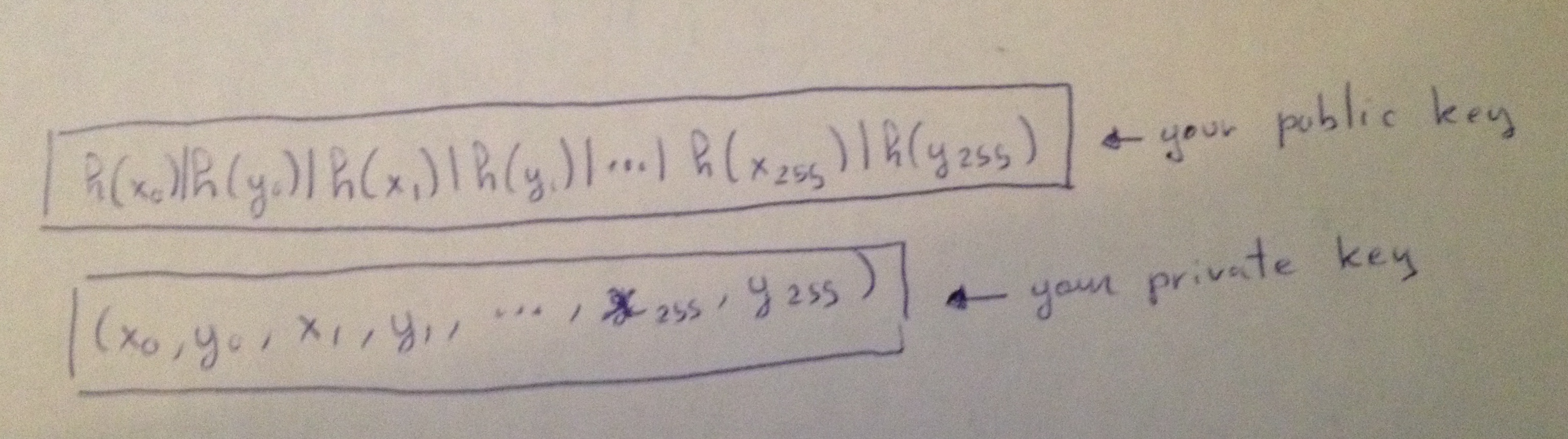
and if you want to sign \(100110_2 \dots\),
you would publish \((y_0,x_1,x_2,y_3,y_4,x_5,\dots)\)
Winternitz OTS (WOTS)
A few months after Lamport's publication, Robert Winternitz of the Stanford Mathematics Department proposed to publish \(h^w(x)\) instead of publishing \(h(x)\|h(y)\).
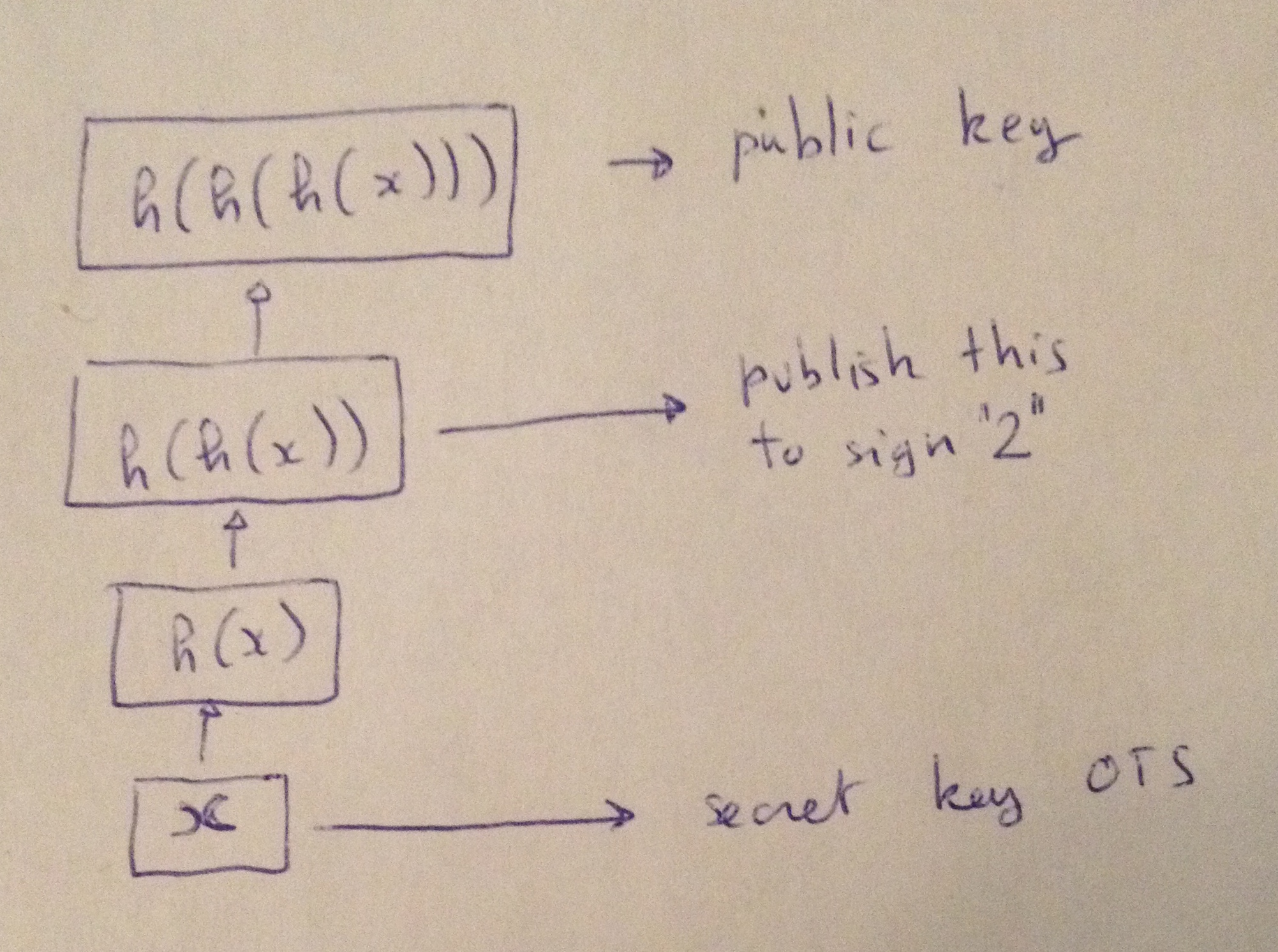
For example you could choose \(w=16\) and publish \(h^{16}(x)\) as your public key, and \(x\) would still be your secret key. Now imagine you want to sign the binary \(1001_2\) (\(9_{10}\)), just publish \(h^9(x)\).
Another problem now is that a malicious person could see this signature and hash it to retrieve \(h^{10}(x)\) for example and thus forge a valid signature for \(1010_2\) (\(10_{10}\)).
This can be circumvented by adding a short Checksum after the message (which you would have to sign as well).
Variant of Winternitz OTS
A long long time after, in 2011, Buchmann et al published an update on Winternitz OTS and introduced a new variant using families of functions parameterized by a key. Think of a MAC.
Now your private key is a list of keys that will be use in the MAC, and the message will dictates how many times we iterate the MAC. It's a particular iteration because the previous output is replacing the key, and we always use the same public input. Let's see an example:
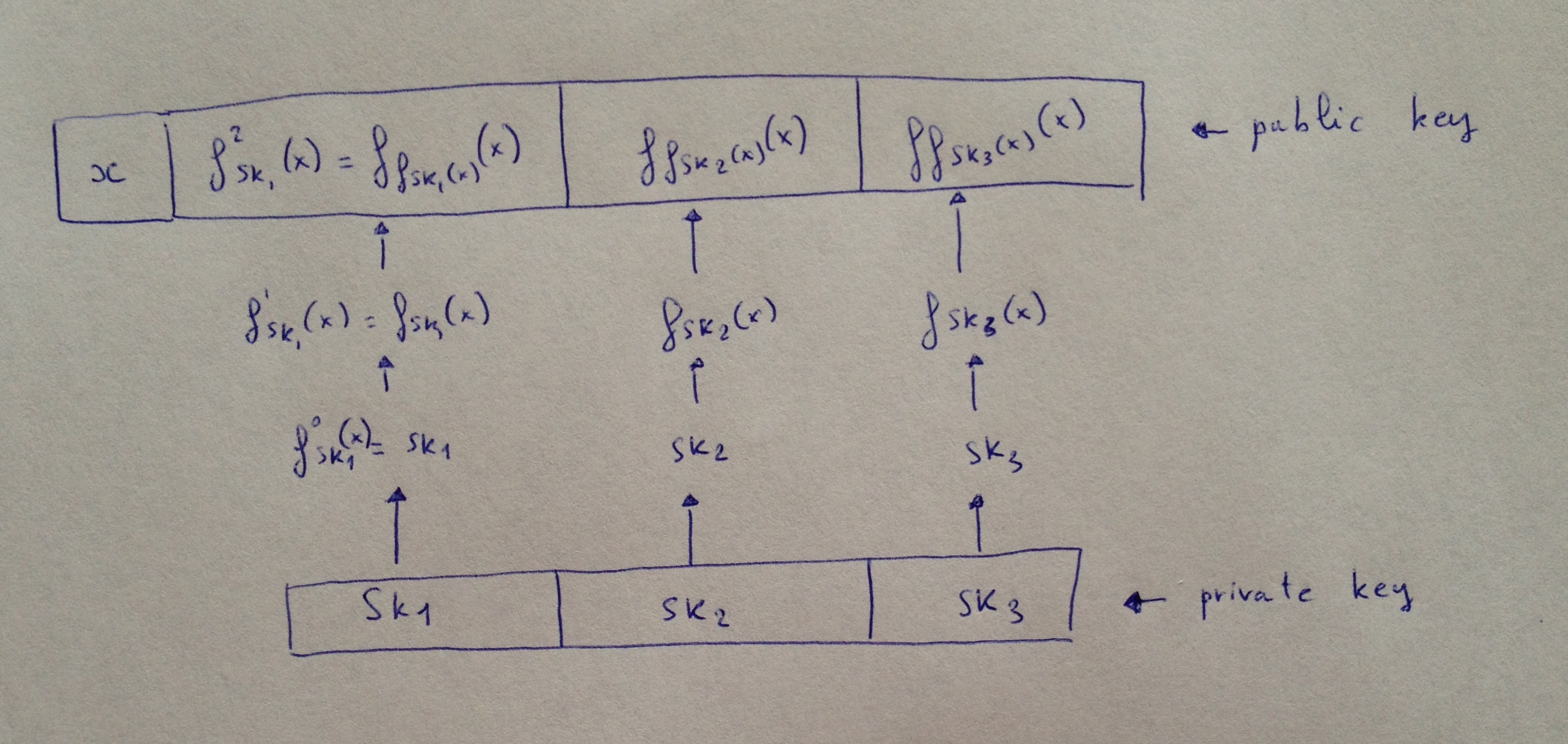
We have a message \(M = 1011_2 (= 11_{10})\) and let's say our variant of W-OTS works for messages in base 3 (in reality it can work for any base \(w\)). So we'll say \(M = (M_0, M_1, M_2) = (1, 0, 2)\) represents \(102_3\).
To sign this we will publish \((f_{sk_1}(x), sk_2, f^2_{sk_3}(x) = f_{f_{sk_3}(x)}(x))\)
Note that I don't talk about it here, but there is still a checksum applied to our message and that has to be signed. This is why it doesn't matter if the signature of \(M_2 = 2\) is already known in the public key.
Intuition tells me that a public key with another iteration would provide better security
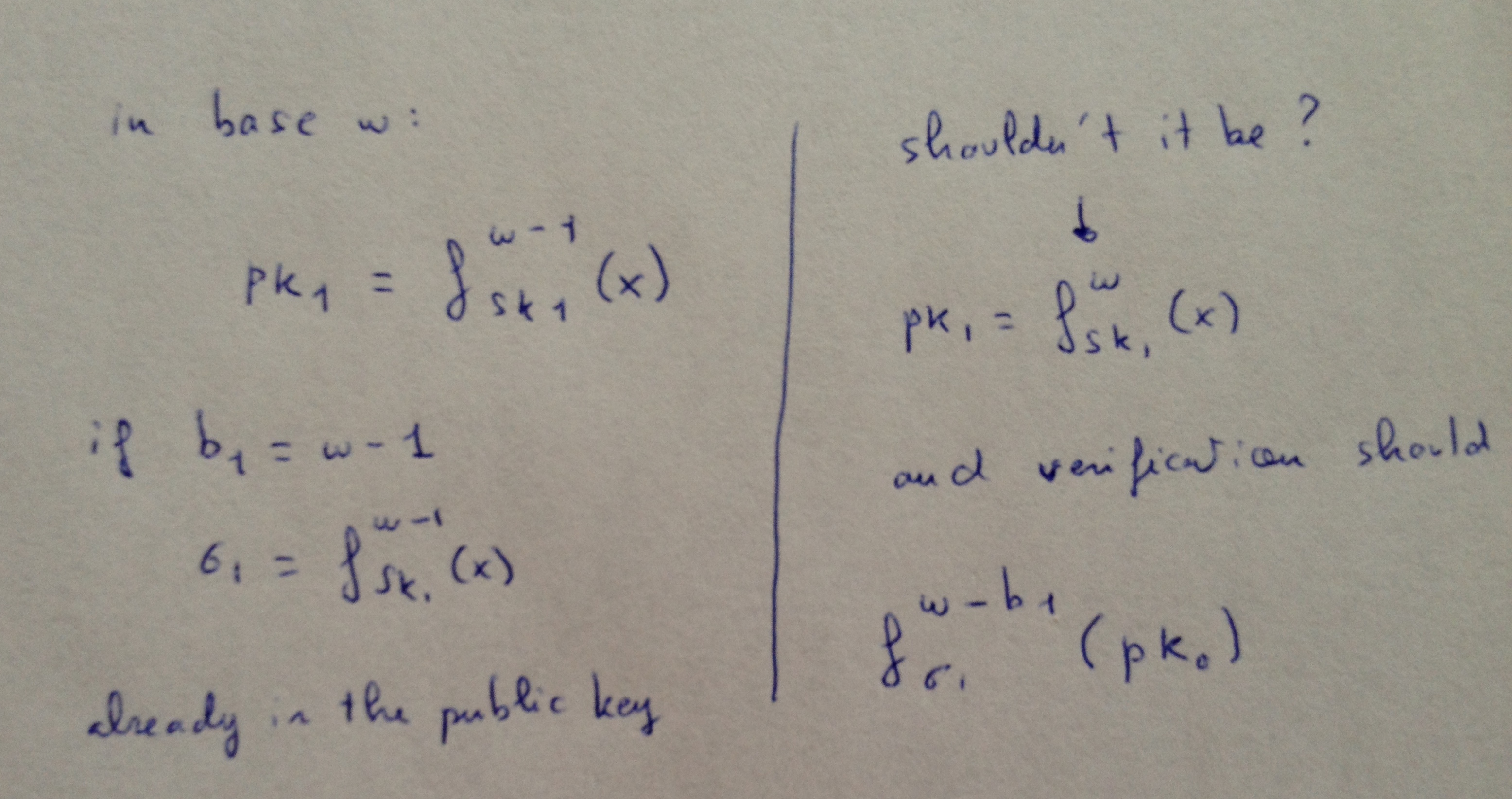
here's Andreas Hulsing's answer after pointing me to his talk on the subject:
Why? For the 1 bit example: The checksum would be 0. Hence, to sign that message one needs to know a preimage of a public key element. That has to be exponentially hard in the security parameter for the scheme to be secure. Requiring an attacker to be able to invert the hash function on two values or twice on the same value only adds a factor 2 to the attack complexity. That's not making the scheme significantly more secure. In terms of bit security you might gain 1 bit (At the cost of ~doubling the runtime).
Winternitz OTS+ (WOTS+)
There's not much to say about the W-OTS+ scheme. Two years after the variant, Hulsing alone published an upgrade that shorten the signatures size and increase the security of the previous scheme. It uses a chaining function in addition to the family of keyed functions. This time the key is always the same and it's the input that is fed the previous output. Also a random value (or mask) is XORed before the one-way function is applied.
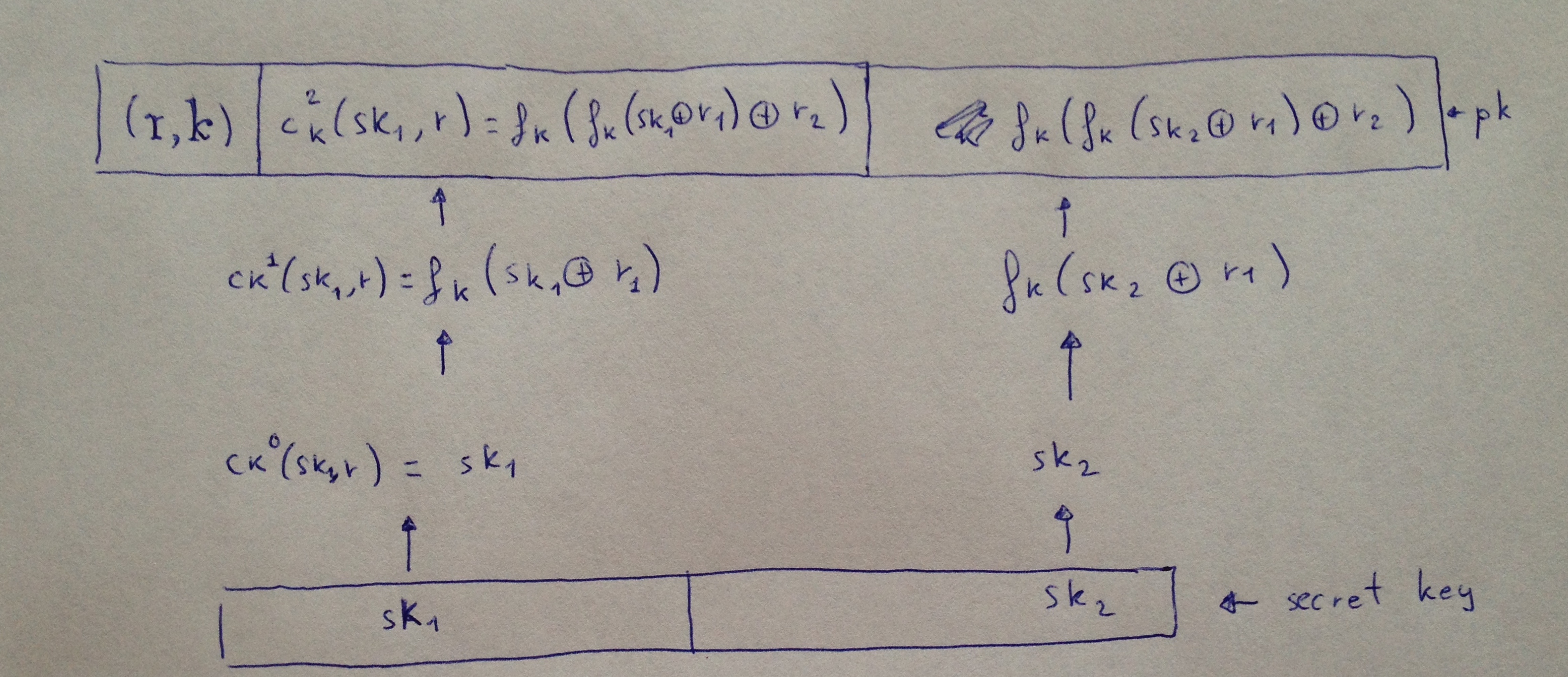
Some precisions from Hulsing about shortening the signatures size:
WOTS+ reduces the signature size because you can use a hash function with shorter outputs than in the other WOTS variants at the same level of security or longer hash chains. Put differently, using the same hash function with the same output length and the same Winternitz parameter w for all variants of WOTS, WOTS+ achieves higher security than the other schemes. This is important for example if you want to use a 128 bit hash function (remember that the original WOTS requires the hash function to be collision resistant, but our 2011 proposal as well as WOTS+ only require a PRF / a second-preimage resistant hash function, respectively). In this case the original WOTS only achieves 64 bits of security which is considered insecure. Our 2011 proposal and WOTS+ achieve 128 - f(m,w) bits of security. Now the difference between WOTS-2011 and WOTS+ is that f(m,w) for WOTS-2011 is linear in w and for WOTS+ it is logarithmic in w.
Other OTS
Here ends today's blogpost! There are many more one-time signature schemes, if you are interested here's a list, some of them are even more than one-time signatures because they can be used a few times. So we can call them few-times signatures schemes (FTS):
So far their applications seems to be reduce to be the basis of Hash-based signatures that are the current advised signature scheme for post quantum usage. See PQCrypto initial recommendations that was released a few months ago.
PS: Thanks to Andreas Hulsing for his comments
Part II of this series is here
What kind of signature should we choose for the future ?
PQCrypto's initial recommendation, published 3 months ago, recommend using hash-based signatures.

Hash-based signatures, or Merkle signatures
The Merkle signature scheme is a digital signature scheme based on hash trees (also called Merkle trees) and one-time signatures such as the Lamport signature scheme. It was developed by Ralph Merkle in the late 1970s and is an alternative to traditional digital signatures such as the Digital Signature Algorithm or RSA.
Reading the SPHINCS whitepaper (a hash-based signature), we can understand a bit more why hash-based signatures were considered by PQCrypto to replace our current quantum weak signatures.
– RSA and ECC are perceived today as being small and fast, but they are broken in polynomial time by Shor’s algorithm. The polynomial is so small that scaling up to secure parameters seems impossible.
– Lattice-based signature schemes are reasonably fast and provide reasonably small signatures and keys for proposed parameters. However, their quanti- tative security levels are highly unclear. It is unsurprising for a lattice-based scheme to promise “100-bit” security for a parameter set in 2012 and to correct this promise to only “75-80 bits” in 2013. Fur- thermore, both of these promises are only against pre-quantum attacks, and it seems likely that the same parameters will be breakable in practice by quantum computers.
– Multivariate-quadratic signature schemes have extremely short signatures, are reasonably fast, and in some cases have public keys short enough for typical applications. However, the long-term security of these schemes is even less clear than the security of lattice-based schemes.
– Code-based signature schemes provide short signatures, and in some cases have been studied enough to support quantitative security conjectures. How- ever, the schemes that have attracted the most security analysis have keys of many megabytes, and would need even larger keys to be secure against quantum computers.
A series of post on hash-based signatures is available here